fetch发起post/put请求
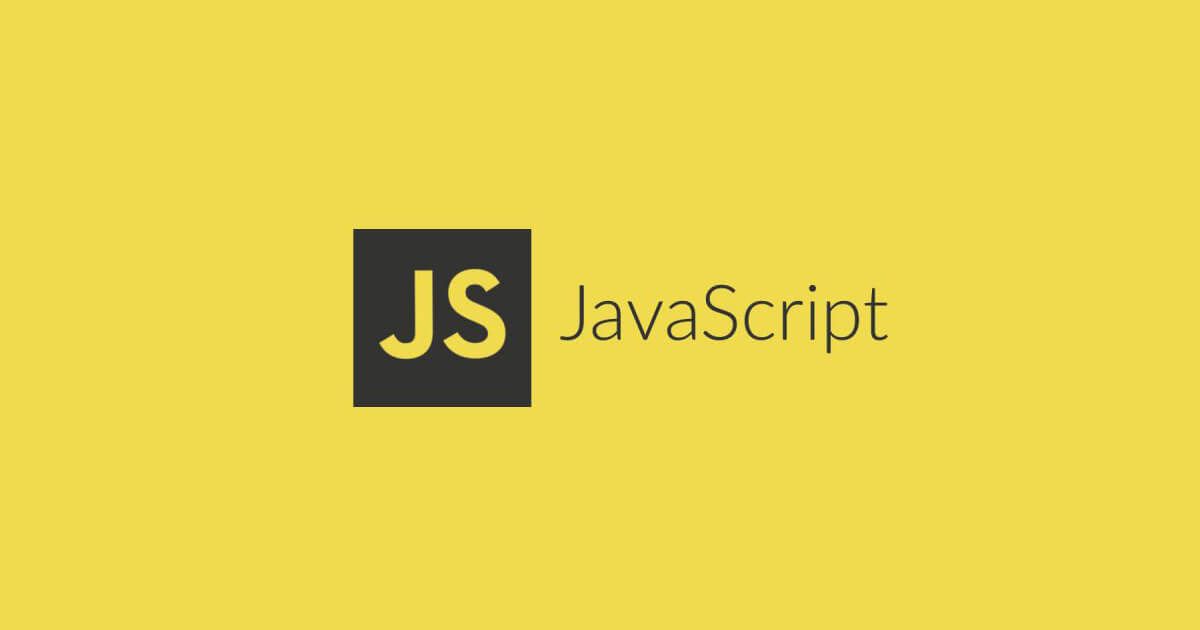
预览
发送json数据
JS1const data = { username: 'example' }; 2 3fetch('https://example.com/profile', { 4 method: 'POST', // or 'PUT' 5 headers: { 6 'Content-Type': 'application/json', 7 }, 8 body: JSON.stringify(data), 9}) 10 .then((response) => response.json()) 11 .then((data) => { 12 console.log('Success:', data); 13 }) 14 .catch((error) => { 15 console.error('Error:', error); 16 });
发送formdata数据
基本使用
JS1// 方式1 2fetch('http://127.0.0.1/api/test', { 3 method: 'POST', 4 headers: { 5 'content-type': 'application/x-www-form-urlencoded', 6 }, 7 body: 'a=1&b=2&c=3', 8}) 9 .then((response) => response.json()) 10 .then((result) => { 11 console.log('Success:', result); 12 }) 13 .catch((error) => { 14 console.error('Error:', error); 15 }); 16 17 18// 方式2 19const body = new FormData(document.getElementById('login-form')); 20fetch('http://127.0.0.1/api/test', { 21 method: 'POST', 22 body, 23});
上传文件
JS1// 单个文件 2function uploadSingleFile() { 3 const formData = new FormData(); 4 const fileField = document.querySelector('input[type="file"]'); 5 6 formData.append('username', 'abc123'); 7 formData.append('avatar', fileField.files[0]); 8 9 fetch('https://example.com/profile/avatar', { 10 method: 'PUT', 11 body: formData, 12 }) 13 .then((response) => response.json()) 14 .then((result) => { 15 console.log('Success:', result); 16 }) 17 .catch((error) => { 18 console.error('Error:', error); 19 }); 20} 21 22// 多个文件 23function uploadMultipleFiles() { 24 const formData = new FormData(); 25 const photos = document.querySelector('input[type="file"][multiple]'); 26 27 formData.append('title', 'title'); 28 for (let i = 0; i < photos.files.length; i++) { 29 formData.append(`photos_${i}`, photos.files[i]); 30 } 31 32 fetch('https://example.com/posts', { 33 method: 'POST', 34 body: formData, 35 }) 36 .then((response) => response.json()) 37 .then((result) => { 38 console.log('Success:', result); 39 }) 40 .catch((error) => { 41 console.error('Error:', error); 42 }); 43}
END
版权声明:本文为博主原创文章,遵循 CC BY-SA 4.0 版权协议,转载请附上原文出处链接和本声明。
本文链接:
分享
打赏
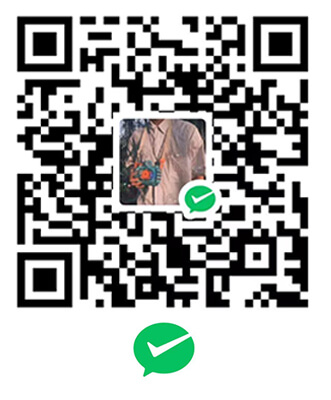
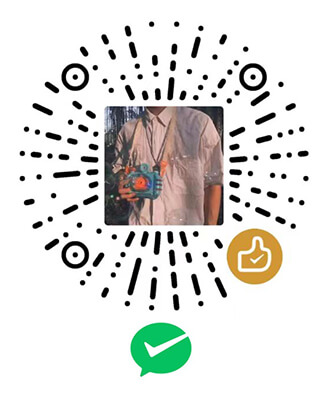
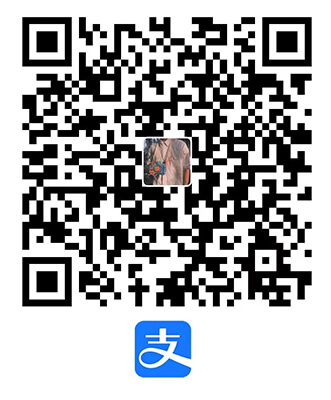
🥰谢谢!!